Sharing Data#
PyProBE makes sharing data simple and straightforward. This is a simple example to demonstrate the process.
First we will import some sample data:
[1]:
%%capture
%pip install matplotlib
[2]:
import os
import shutil
from pprint import pprint
import pyprobe
%matplotlib inline
# Describe the cell. Required fields are 'Name'.
info_dictionary = {
"Name": "Sample cell",
"Chemistry": "NMC622",
"Nominal Capacity [Ah]": 0.04,
"Cycler number": 1,
"Channel number": 1,
}
# Create a cell object
cell = pyprobe.Cell(info=info_dictionary)
data_directory = "../../../tests/sample_data/neware"
cell.add_procedure(
procedure_name="Sample",
folder_path=data_directory,
filename="sample_data_neware.parquet",
)
We can then use the archive()
method of the cell object. This stores all attributes of the cell
object into a single folder. The data is stored as .parquet
files and the metadata is stored in .json
files.
[3]:
cell.archive(path="sample_archive")
os.listdir(".")
[3]:
['ocv-fitting.ipynb',
'sample_archive',
'comparing-pyprobe-performance.ipynb',
'sharing-data.ipynb',
'differentiating-voltage-data.ipynb',
'filtering-data.ipynb',
'getting-started.ipynb',
'examples.rst',
'working-with-pybamm-models.ipynb',
'providing-valid-inputs.ipynb',
'analysing-GITT-data.ipynb',
'plotting.ipynb']
You can choose to compress the folder by adding .zip
to the path:
[4]:
cell.archive(path="sample_archive.zip")
os.listdir(".")
[4]:
['ocv-fitting.ipynb',
'comparing-pyprobe-performance.ipynb',
'sharing-data.ipynb',
'differentiating-voltage-data.ipynb',
'sample_archive.zip',
'filtering-data.ipynb',
'getting-started.ipynb',
'examples.rst',
'working-with-pybamm-models.ipynb',
'providing-valid-inputs.ipynb',
'analysing-GITT-data.ipynb',
'plotting.ipynb']
You can then retrieve the archived object with the pyprobe.load_archive()
method:
[5]:
saved_cell = pyprobe.load_archive("sample_archive.zip")
pprint(saved_cell.info)
{'Channel number': 1,
'Chemistry': 'NMC622',
'Cycler number': 1,
'Name': 'Sample cell',
'Nominal Capacity [Ah]': 0.04}
[6]:
saved_cell.procedure["Sample"].plot(x="Time [hr]", y="Voltage [V]")
[6]:
<Axes: xlabel='Time [hr]'>
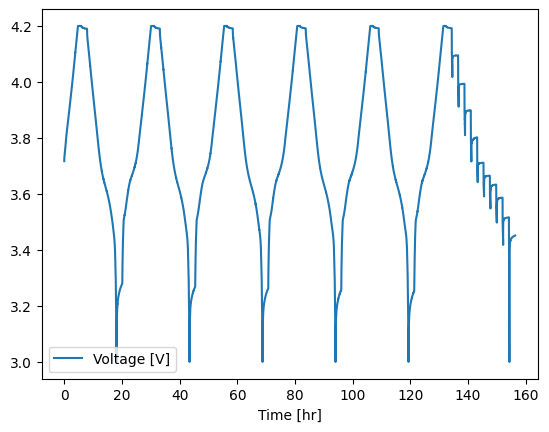
Clean up after example
[7]:
shutil.rmtree("sample_archive")