Plotting#
PyProBE includes compatibility with multiple open plotting libraries in Python. This example will go through interacting with each of them.
Start by importing PyProBE and the dataset:
[1]:
%%capture
%pip install matplotlib
[2]:
import pyprobe
import matplotlib.pyplot as plt
%matplotlib inline
[3]:
info_dictionary = {
"Name": "Sample cell",
"Chemistry": "NMC622",
"Nominal Capacity [Ah]": 0.04,
"Cycler number": 1,
"Channel number": 1,
}
data_directory = "../../../tests/sample_data/neware"
# Create a cell object
cell = pyprobe.Cell(info=info_dictionary)
cell.add_procedure(
procedure_name="Sample",
folder_path=data_directory,
filename="sample_data_neware.parquet",
)
Pandas/Matplotlib#
Matplotlib is one of the most popular plotting libraries in Python. Pandas dataframes include a built-in plot()
method to construct plots using Matplotlib, which is also integrated into PyProBE Result
objects.
Call plot()
on any result object and control the method exactly as you would expect to with Pandas:
[4]:
plt.figure()
cell.procedure["Sample"].experiment("Break-in Cycles").plot(
x="Time [s]", y="Voltage [V]", grid=True
)
[4]:
<Axes: xlabel='Time [s]'>
<Figure size 640x480 with 0 Axes>
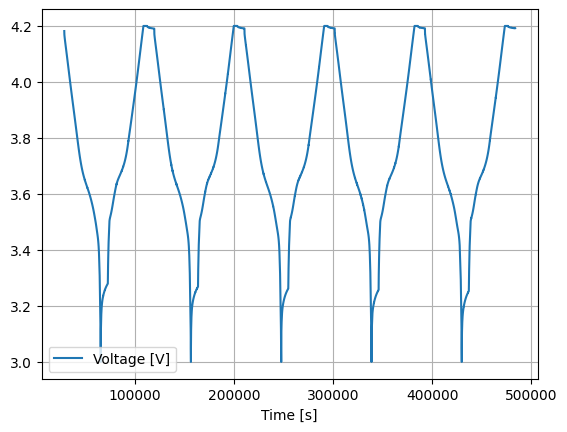
HvPlot#
Plots created using Matplotlib sometimes need a lot of work to look good, and they are not particularly interactive. HvPlot provides a high-level interface similar to plotting in pandas with the additional flexibility of specifying a more interactive backend. Note hvplot is an optional dependency, it can be installed seperately to enable this functionality.
[5]:
cell.procedure["Sample"].experiment("Break-in Cycles").hvplot(
x="Time [s]", y="Voltage [V]"
)
[5]: